環境
$ uname -a
Linux ps2 5.10.63-v7l+ #1457 SMP Tue Sep 28 11:26:14 BST 2021 armv7l GNU/Linux
$ cat /etc/os-release
PRETTY_NAME="Raspbian GNU/Linux 10 (buster)"
NAME="Raspbian GNU/Linux"
VERSION_ID="10"
VERSION="10 (buster)"
VERSION_CODENAME=buster
ID=raspbian
ID_LIKE=debian
インストールの参考にしたサイト
「Raspberry Pi 3B+における、Tesseract(5.0.0 alpha)のインストール方法と基本操作」
$ sudo apt-get install tesseract-ocr-script-jpan
$ tesseract --version
tesseract 4.0.0
leptonica-1.76.0
libgif 5.1.4 : libjpeg 6b (libjpeg-turbo 1.5.2) : libpng 1.6.36 : libtiff 4.1.0 : zlib 1.2.11 : libwebp 0.6.1 : libopenjp2 2.3.0
$ tesseract --list-langs
List of available languages (3):
Japanese
eng
osd
日本語のトレーニングデータ取得とインストール(精度優先)
$ git clone https://github.com/tesseract-ocr/tessdata_best.git
$ sudo cp tessdata_best/jpn* /usr/share/tesseract-ocr/4.00/tessdata/
追加したトレーニングデータの確認
$ tesseract --list-langs
List of available languages (5):
Japanese
eng
jpn
jpn_vert
osd
ペイントプログラムで書いたTEST-JPN.pngファイを認識させてみる。
フォントサイズ(20/12)と書体(BOLD)の違いも確認。
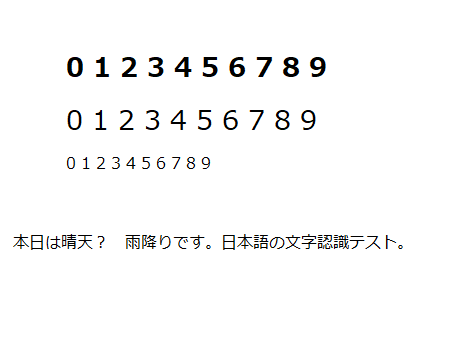
$ time tesseract TEST-JPN.png stdout -l jpn
0123456789
0123456789
0123456789
本 日 は 晴天 ? 雨降り で す 。 日 本 語 の 文字 認識 テス ト 。
real 1m4.283s
user 1m43.622s
sys 0m3.248s
この例では、なんと認識率100%。
罫線の中の数字をも試してみました。(TEST-JPN3.png)
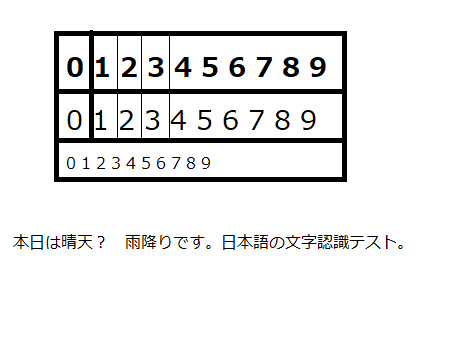
表組にすると、まったく認識されない結果に!
$ time tesseract TEST-JPN3.png stdout -l jpn
Empty page!!
Empty page!!
real 0m4.568s
user 0m2.977s
sys 0m1.382s
表を認識させる記事があったので、こちらも試してみたが、pytesseract.image_to_stringを呼び出した後で、戻ってこない印象。 戻ってきていますが、空文字列となって、認識に失敗しているようです。前述のコマンドラインで認識できた画像は、pythonのコードでも問題なく認識。
「『表の文字』と『欄外の文字』の認識(Python + Tesseract)」
ここから、jupyter notebookで pytesseract をインストール
!pip install pytesseract
Looking in indexes: https://pypi.org/simple, https://www.piwheels.org/simple
Collecting pytesseract
Downloading https://www.piwheels.org/simple/pytesseract/pytesseract-0.3.8-py2.py3-none-any.whl (14 kB)
Requirement already satisfied: Pillow in /home/mars/.pyenv/versions/3.7.3/lib/python3.7/site-packages (from pytesseract) (8.3.1)
Installing collected packages: pytesseract
Successfully installed pytesseract-0.3.8
認識のテストコード
###############################################################################
# ライブラリインポート
###############################################################################
import os # os の情報を扱うライブラリ
import pytesseract # tesseract の python 用ライブラリ
from PIL import Image # 画像処理ライブラリ
import matplotlib.pyplot as plt # データプロット用ライブラリ
import numpy as np # データ分析用ライブラリ
img=Image.open('/home/mars/TEST-JPN3.png')
# 画像を配列に変換
im_list = np.array(img)
# データプロットライブラリに貼り付け
plt.imshow(im_list)
# 表示
plt.show()
print('Start....')
# テキスト抽出
txt = pytesseract.image_to_string(img,lang="jpn")
# 抽出したテキストの出力
print('Done.')
print(txt)
print()
枠線を消す処理を追加
import cv2
from PIL import Image # 画像処理ライブラリ
from matplotlib import pyplot as plt # データプロット用ライブラリ
import numpy as np # データ分析用ライブラリ
import os # os の情報を扱うライブラリ
import pytesseract # tesseract の python 用ライブラリ
# 処理の対象
img = cv2.imread("/home/mars/TEST-JPN4.png")
img2 = img.copy()
img3 = img.copy()
# グレースケール
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray_list = np.array(gray)
# データプロットライブラリに貼り付け
#plt.imshow(gray_list)
#cv2.imwrite("calendar_mod.png", gray)
## 反転 ネガポジ変換
gray2 = cv2.bitwise_not(gray)
gray2_list = np.array(gray2)
#plt.imshow(gray2_list)
#cv2.imwrite("calendar_mod2.png", gray2)
lines = cv2.HoughLinesP(gray2, rho=1, theta=np.pi/360, threshold=80, minLineLength=80, maxLineGap=5)
for line in lines:
x1, y1, x2, y2 = line[0]
# 赤線を引く
red_lines_img = cv2.line(img2, (x1,y1), (x2,y2), (0,0,255), 3)
red_lines_np=np.array( red_lines_img)
#cv2.imwrite("calendar_mod3.png", red_lines_img)
# 線を消す(白で線を引く)
no_lines_img = cv2.line(img3, (x1,y1), (x2,y2), (255,255,255), 3)
no_lines=np.array( no_lines_img)
plt.imshow(no_lines)
#plt.show()
#cv2.imwrite("calendar_mod4.png", no_lines_img)
print('OCR start...')
#txt = pytesseract.image_to_string(img, lang="jpn",config='osd --psm 6')
txt = pytesseract.image_to_string(no_lines_img, lang="jpn",config='--psm 6')
plt.show()
#cv2.imwrite("/home/mars/line_erased.png",no_lines_img)
print('---------OCR:results-----')
print(txt)
print('---------OCR done.---------')
線が消された画像
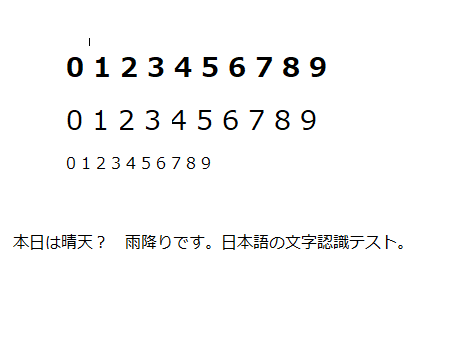
認識結果。問題なく認識。ただし、以上は理想的(?)にきれいな画像の場合。カメラで撮影した画像については、さらに検証や処理の追加が必要になるかも??
---------OCR:results-----
0123456789
0123456789
0123456789
本 日 は 晴天 ? 雨降り で す 。 日 本 語 の 文字 認識 テス ト 。
---------OCR done.-------